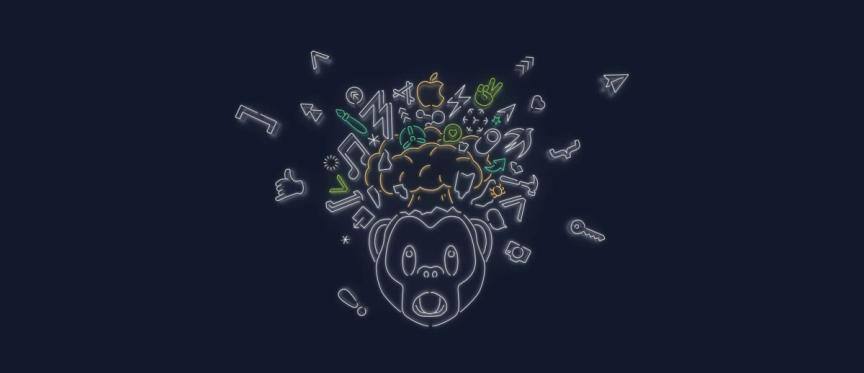
In the world of iOS development, Apple's Combine framework is gaining more and more popularity due to its flexibility and ease of use. Last week, we delved into some key ideas of the Combine framework, including Publishers, Operators, and key-path Bindings. Today, we're going to take a deeper look into another important concept of Combine: Subscribers and Subjects.
Subscribers
In the previous post, we took a brief look at Subscribers. We implemented key-path binding Subscriber. Now we can check another type of Subscribers in the Combine framework, Sinks. Sink Subscriber assigns the closure to the output stream, and as soon as new value appears, it runs closure and passes a new value as a parameter. Let’s take a look at the code samples.
private func fetchRepos(matching query: String) {
githubService
.search(matching: query)
.replaceError(with: [])
.subscribe(on: DispatchQueue.global())
.receive(on: OperationQueue.main)
.sink { repos in print("count:", repos.count) }
}
As you can see in the example above, we use the sink operator to create a Subscriber type which runs closure as soon as new values are published.
Subjects
Another interesting type of Subscriber provided by Combine framework is Subjects. Subjects can behave simultaneously as a Publisher and Subscriber. You can create a Subject instance to send some value and subscribe on it. Here is a simple example.
let subject = CurrentValueSubject<String, Never>("Hello")
subject.sink { value in print(value) }
subject.send("World")
The Combine framework provides two types of Subjects: CurrentValueSubject and PassthroughSubject. The only difference between them is value caching. PassthroughSubject publishes only values which appear after subscription, while CurrentValueSubject holds the latest value, and as soon as Subscriber assigned to Subject, it receives the most recent value. To understand the difference between them, try to replace CurrentValueSubject with PassthroughSubject and run the example.
@Published
Swift 5.1 released a new language feature called Property Wrappers. This allows you to easily wrap properties with any custom logic. More about Property Wrappers can be read in the proposal. Combine framework has a special Property Wrapper, which can be used to convert any property to a Publisher.
@Published private var query: String = ""
private func fetchRepos(matching query: String) {
$query.flatMap {
self.githubService
.search(matching: $0)
.replaceError(with: [])
}
.subscribe(on: DispatchQueue.global())
.receive(on: OperationQueue.main)
.assign(to: \.repos, on: self)
}
Schedulers
Combine provides a nice way to control delivery and work queues. Often, we want to move a value assigned to the main-thread because of the User Interface rendering process. Combine provides the receive operator, which is used to control receiving (delivery) queue. Similarly, we have the subscribe operator, which we can use to move the value publishing process onto a specific queue. Let’s take a look at the code example:
private func fetchRepos(matching query: String) {
githubService
.search(matching: query)
.replaceError(with: [])
.subscribe(on: DispatchQueue.global())
.receive(on: OperationQueue.main)
.assign(to: \.repos, on: self)
}
Both receive and subscribe operators accept DispatchQueue or OperationQueue as parameters to specify a queue.
Debugging
There is a known problem in using FRP frameworks, which is difficulties during the debugging of huge Publisher chains. Combine framework provides several operators to make debugging a bit easier. You can add a call to print operator in a Publisher chain. The “Print” operator will print every event in the chain to your Xcode console. Another useful tool for debugging can be a Breakpoint operator. It causes debugger signal which you can use to inspect an event in your debugger console.
Conclusion
Today we discussed some more key concepts of Combine framework. It’s time to convert your asynchronous logic into Publishers. Feel free to contact me to ask your questions about the Combine framework. Thanks for reading!