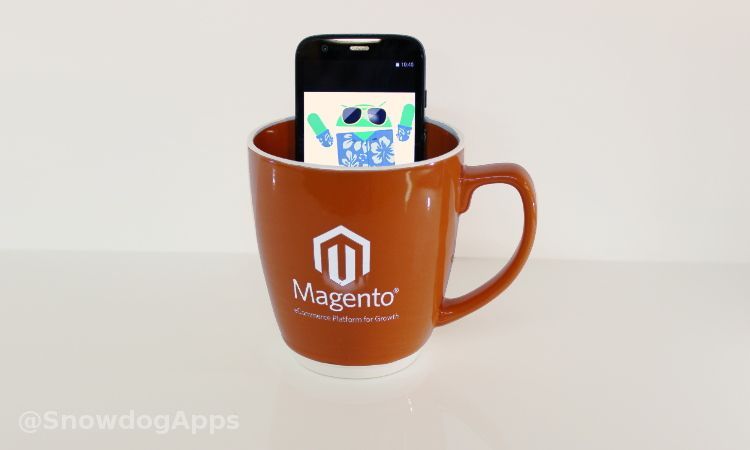
Use Retrofit with Magento SOAP API
Today we will use REST client Retrofit to communicate with Magento SOAP API. There is a bit of code to write at beginning which seems to be a high entry barrier to overcome. But look below – I've written everything :)
The brief about SOAP
Magento SOAP API requires XML messages which consist of Envelope XML element and Body inside Envelope. Operation to perform will be another element inside Body named in definition of API (WSDL file http://magentohost/api/v2_soap?wsdl=1 ); parameters are nested in Operation. All requests in Magento SOAP API are performed via POST at http://magentohost/api/v2_soap so Operation name define what action will be performed.
Project setup
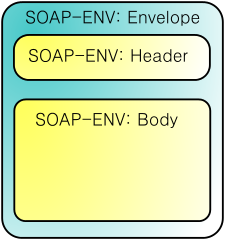
First add necessary dependencies into build.gradle
Define model
There is Envelope with defined namespaces and body. We use SimpleXml annotations to match XML elements and attributes with Java fields. SimpleXml website contains a lot of useful tutorials. You will be really thankful to their authors, when you get to serialization of complex lists of objects.
RequestBody class with “object” field is container for input message. We use @Element annotation with name parameter to control which operation will be called. If you call other operation then just change the name programmatically during serialization.
Login input contains username and apiKey. API user must be defined in Magento backend (look under System > Web Services > SOAP/XML-RPC Usesrs).
Output is very simple and should contain valid response field loginReturn (session id). It is required to make every other API call to download product list, details, images and so on.
RestAdapter and API interface
We define asynchronous login method in MagentoApi interface. Note that there defined another method for downloading list of products. It differs from REST API because all operations use the same endpoint and only RequestBody and Callback response type are changed.
Next we build RestAdapter with SimpleXMLConverter and basic AnnotationStrategy. LogLevel.FULL is very useful for debugging.
Define Callback and make a call
We have got code base and are ready to develop our Magento Store application. Of course there is more code than in previous approach but it will be used to other requests. SimpleXml works pretty well with Retrofit and (de)serialize all models. Magento API documentation describes how it works but if something doesn’t work like docs says check out WSDL file and server response (Retrofit displays all logs).
In next post I will show you how to handle errors of communication with Magento SOAP API.